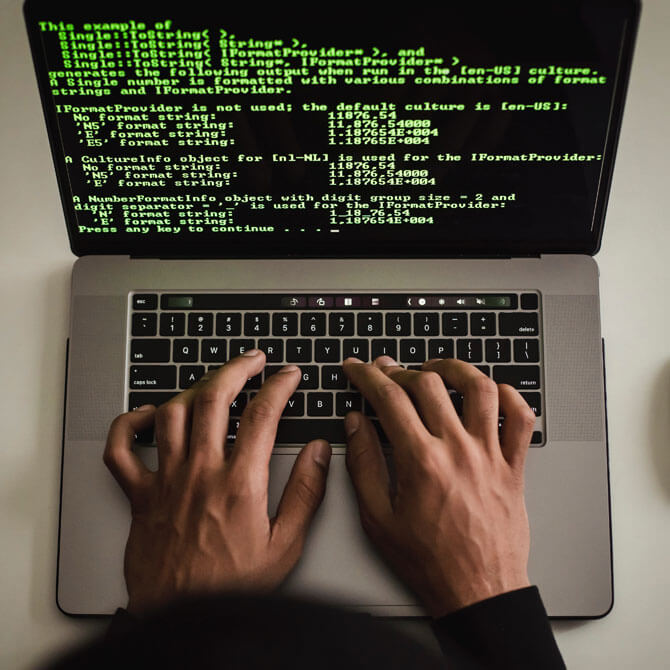
Background
As a computer engineering major at Montgomery College and UMD, I worked with C++, C#, Java, Ruby, Pascal, Verilog, and assembly code. More recently, I have been focused on developing tools with Python and Powershell scripts, but also spend time during CTF’s reverse engineering C and assembly in Ghidra and EDB. I am experienced enough to understand most of what I see in the wild and efficiently cobble together programs through expert adoption of open-source repositories.
In Progress
Name: EZVulnFinder
Language: Python
Function: Port scanner that automatically runs discovered services through vulnerability databases and returns suggestions for potential attack vectors.
FlagDumper - Python
#!/user/bin/env python3
# FlagDumper.py
## Checks for low-hanging fruit for network forensics challenges in CTF's!
## Automatically searches for the ascii and hex representation of "flag"
## Additionally searches for ascii and hex representation of strings given by user
import os
import binascii
# variable declaration
file_or_dir = ""
special = ""
special_exists = ""
checks = ["flag"] ## keywords to search for
## initial prompts
while (special_exists != "y") and (special_exists != "n"):
special_exists = input(
"Would you like to enter a flag precursor other than \"flag\" (ex: THM{, HTB{, etc.)? (y/n):")
if (special_exists == "y"):
special = input("Please enter flag precursor:")
checks.append(special)
while (file_or_dir != "single") and (file_or_dir != "directory"):
file_or_dir = input("Analyzing a single capture, or directory of captures? (single/directory):")
def analyze_capture(location):
for x in checks:
x_enc = binascii.hexlify(x.encode()).decode("utf-8")
cmd = 'tshark -r ' + location + ' -Y "frame contains \\"' + x + '\\"" -T fields -e data | xxd -r -p' # Check for ascii representation
cmd_enc = 'tshark -r ' + location + ' -Y "frame contains \\"' + x_enc + '\\"" -T fields -e data | xxd -r -p | xxd -r -p' # Check for Hex representation
os.system(cmd)
os.system(cmd_enc)
if (file_or_dir == "single"):
location = input("Please Enter Capture Location:\n")
analyze_capture(location)
else:
location = input("Please Enter Directory:\n")
for filename in os.listdir(location):
print(filename + ": ")
analyze_capture((location + filename))
print("\n")
Connection Detector - Powershell
I designed this for CCDC to establish a baseline of network connections, then display any new connections that weren’t there when we started.
#Create baseline
netstat -ano |Out-File -FilePath .\baseline.txt
Copy-Item ".\baseline.txt" -Destination ".\current.txt"
#Check Connections
$trip = 0
$a = netstat -ano
$a[6..$a.count] | ConvertFrom-String | select p4 |Out-File -FilePath .\temp.txt
gc .\temp.txt | sort | get-unique > .\temp1.txt
$regexIPAddress = '\b\d{1,3}\.\d{1,3}\.\d{1,3}\.\d{1,3}\b.'
echo "******************************** BRAND NEW CONNECTIONS ********************************"
foreach($line in Get-Content .\temp1.txt) {
if($line -match $regexIPAddress){
if($line -ne ""){
if($line -like "*4444*"){
$trip++
}
if($trip -eq 1){
[System.Windows.Forms.MessageBox]::Show('Malicious connection suspected!')
}
$SEL = Select-String -Path .\current.txt -Pattern $line
if ($SEL -eq $null)
{
echo $line
}
}
}
}
echo "******************************** OTHER NEW CONNECTIONS ********************************"
foreach($line in Get-Content .\current.txt) {
if($line -match $regexIPAddress){
if($line -ne ""){
$SEL = Select-String -Path .\baseline1.txt -Pattern $line
if ($SEL -eq $null)
{
echo $line
}
}
}
}
Copy-Item ".\temp1.txt" -Destination ".\current.txt"